Understanding Responsive Web Pages: A Comprehensive Guide
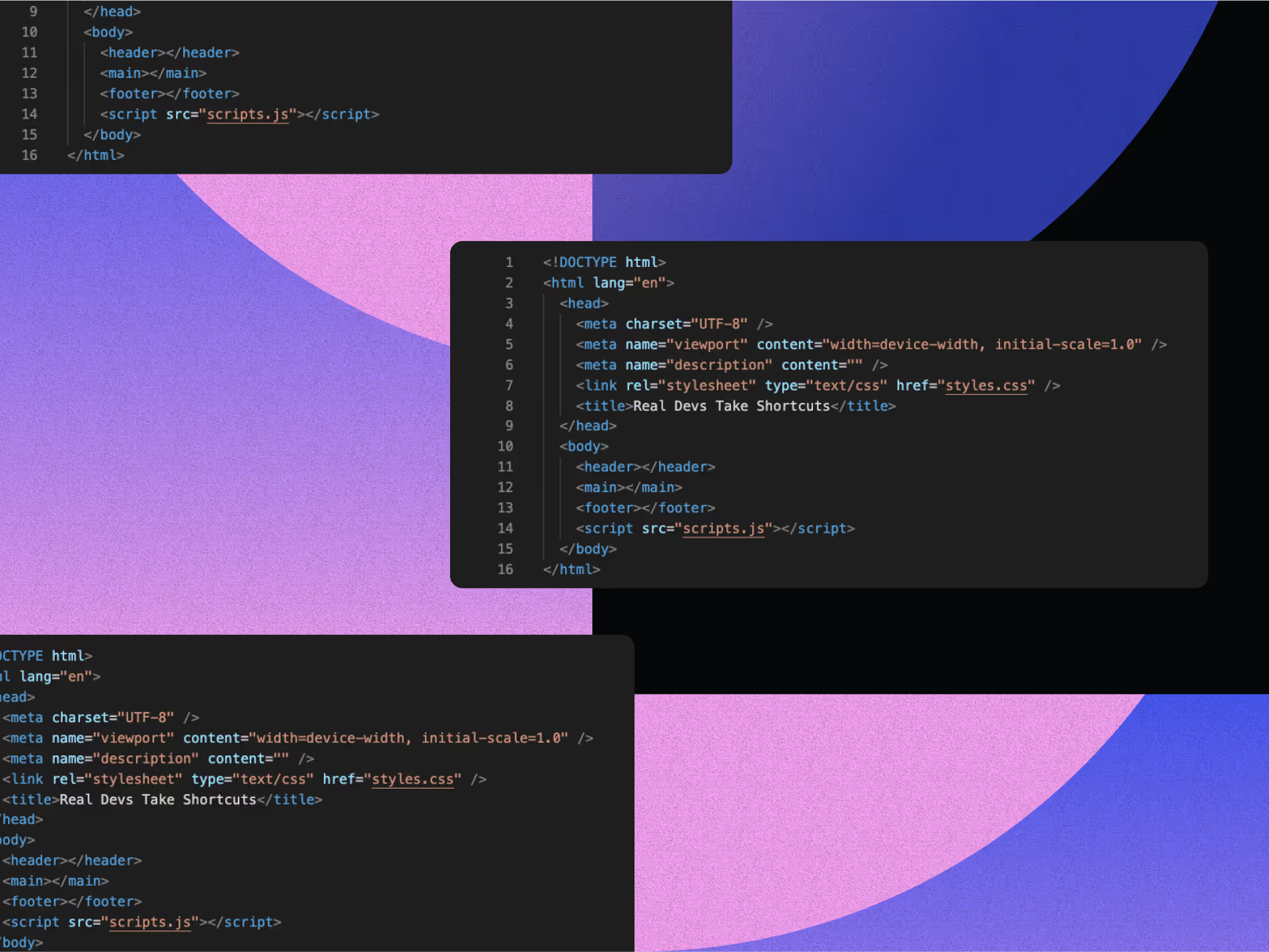
Your website visitors aren't just sitting at desktops anymore. They're scrolling on phones during commutes, tapping tablets while lounging on couches, hammering away on laptops at bustling cafés, and clicking through desktops at the office.
Understanding responsive web pages is crucial. After all, responsive and mobile-first web design aren't just lovely to have either; they're the backbone of brilliant digital experiences, automatically adjusting to deliver magic across all these devices.
We've waved goodbye to those clunky "mobile versions" of websites. Now, responsive design is simply how we breathe life into the web. When done right, it banishes the frustration of pinching, zooming, or side-scrolling just to see what's on screen. This article provides you with a guide on how responsive web pages work.
The Fundamentals of Responsive Web Pages
HTML naturally flows, with text wrapping based on available space. However, actual responsive design, where layouts adapt seamlessly to different screen sizes, requires specific CSS techniques.
The approach is built on three core ideas first defined by Ethan Marcotte in 2010: fluid grids, flexible images, and media queries. These work together so layouts adapt to different screens without changing the content.
Master these basics, and you'll have what you need for responsive design, no matter which specific CSS methods you use. The key? Design for flexibility, not fixed dimensions, is a principle central to any web design checklist.
The Viewport Meta Tag: The Foundation of Mobile Responsiveness
Before you write a single line of CSS, there's one tiny-but-mighty addition your HTML desperately needs: the viewport meta tag. This little powerhouse completely transforms how mobile browsers handle your site.
Skip this tag, and mobile browsers assume your page is built for desktop. They'll render it at a default width (usually 980px), then shrink it to fit mobile screens. The result? Tiny, squint-inducing text that forces users to zoom and pan around like they're navigating a digital maze.
Here's what you need:
<meta name="viewport" content="width=device-width, initial-scale=1">
This tells mobile browsers to set the viewport width to match the device width and show content at a 1:1 scale. According to Google’s PageSpeed Insights and other tools like the Mobile-Friendly Test, sites without a proper viewport setting are flagged as not mobile-friendly. This can hurt user experience and negatively impact search rankings.
Fluid Layouts and Relative Units
Kiss those rigid pixel dimensions goodbye! Responsive layouts embrace relative units that flex and flow based on screen size. This lets your content breathe naturally within the available space.
Instead of:
.container {
width: 960px;
}
Use percentage-based widths:
.container {
width: 90%;
max-width: 1200px;
margin: 0 auto;
}
This creates a container that is 90% of the parent element's width (usually the viewport) but never grows beyond 1200px. The margin values center it horizontally.
For text and spacing, em units (relative to the parent element) or rem units (relative to the root element), which scale proportionally:
body {
font-size: 16px; /* Base size */
}
h1 {
font-size: 2rem; /* Always 2× the root font size */
margin-bottom: 1.5rem;
}
Viewport units (vw/vh) offer another option, sizing elements relative to viewport dimensions rather than parent elements.
Understanding Responsive Web Pages: Modern CSS Layout Methods for Responsive Design
Remember the dark days of responsive design? Those complex float-based grids with endless clearfix solutions? Layouts needed mountains of code to prevent unexpected wrapping and maintain spacing across devices.
Today's CSS gives us intuitive, powerful layout methods that are responsive by nature, making building responsive websites easier than ever. These fresh approaches create adaptable layouts with less code and fewer media queries while giving you superhero-level control over element positioning and behavior.
Flexbox in Responsive Web Pages: Creating Flexible Content Rows and Columns
Flexbox isn't just good; it's a game-changer that arranges elements in a single row or column. It's perfect for website navigation, card layouts, and form elements that need to adapt to different screens.
Usage statistics from StatCounter show that most web users are on browsers that support Flexbox. According to Can I Use, over 98% of browsers now support Flexbox, so in most cases, you can use it without worrying about fallbacks.
Here's a responsive navigation that transforms from horizontal on desktop to vertical on mobile:
.nav {
display: flex;
flex-wrap: wrap; /* Allow items to wrap on smaller screens */
}
.nav-item {
flex: 0 1 auto; /* Don't grow, allow shrinking, auto basis */
}
@media (max-width: 768px) {
.nav {
flex-direction: column; /* Stack vertically on mobile */
}
}
Flexbox shines with properties like justify-content (alignment along the central axis) and align-items (alignment along the cross axis), creating layouts that adapt to available space.
CSS Grid in Responsive Web Pages: Building Responsive Page Structures
While Flexbox handles one-dimensional layouts, CSS Grid gives you a two-dimensional system for complex page structures. Grid lets you control both rows and columns at once.
The fr unit (fractional unit) shares available space proportionally:
.grid-container {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(250px, 1fr));
gap: 20px;
}
This creates a responsive grid where each column is at least 250px wide, and columns adjust automatically to fill available space. As the screen narrows, columns wrap to the next row without needing media queries.
Rachel Andrew's grid research shows that CSS Grid cuts down the code needed for complex responsive layouts while giving better control over element placement. For nested components, use Grid for the overall page structure and Flexbox for individual elements, playing to each tool's strengths and enhancing user experience with micro-interactions.
How to Tailor Designs to Different Devices in Responsive Web Pages
Media queries are the secret sauce that lets you apply CSS rules based on device characteristics like screen width, height, or orientation. They're what allow responsive web pages to change layouts dramatically across devices.
The basic syntax targets specific screen width ranges:
/* Styles for screens 768px and wider */
@media (min-width: 768px) {
.container {
padding: 2rem;
}
}
Many developers prefer a mobile-first approach, writing base styles for small screens and then using media queries to enhance the layout for larger displays, optimizing user experience. This typically creates more efficient code and better performance on mobile devices.
/* Base styles for all screens (mobile first) */
.card-container {
display: flex;
flex-direction: column;
}
/* Enhanced layout for tablets and larger */
@media (min-width: 768px) {
.card-container {
flex-direction: row;
flex-wrap: wrap;
}
.card {
flex: 0 0 calc(50% - 1rem);
}
}
/* Further enhancements for desktop */
@media (min-width: 1200px) {
.card {
flex: 0 0 calc(33.333% - 1rem);
}
}
Standard Breakpoints and Best Practices in Understanding Responsive Web Pages
Forget targeting specific devices, focus on breakpoints where your content naturally needs adjustment. That said, these standard ranges work wonderfully as starting points:
- Small devices: up to 600px
- Medium devices: 600px to 900px
- Large devices: 900px to 1200px
- Extra-large devices: 1200px and above
Luke Wroblewski’s writing and expertise emphasize the importance of accessible, responsive design. Using relative units like em for breakpoints is a widely accepted best practice that improves accessibility by allowing layouts to respond to user font size preferences.
@media (min-width: 48em) { /* 768px at default font size */ }
Test layouts at various widths between breakpoints, not just at specific breakpoint sizes. Designs should work smoothly across the entire range of possible screen sizes.
Keep media queries organized in your CSS, grouped by component or collected near the end of your stylesheet, to maintain readability as projects grow.
Media Query Features Beyond Width
While width-based queries are most common, other media features can enhance responsive designs:
/* Apply styles in landscape orientation */
@media (orientation: landscape) {
.hero {
height: 70vh;
}
}
/* Apply styles only on devices that support hover */
@media (hover: hover) {
.card:hover {
transform: translateY(-5px);
}
}
/* Handle high-resolution displays */
@media (min-resolution: 2dppx) {
.logo {
background-image: url('logo@2x.png');
}
}
For touch devices where hover isn't available, Microsoft’s research and design guidelines recommend larger touch targets, typically at least 40px × 40px or 44px × 44px, and different interaction patterns than those used for mouse-based interfaces. This approach improves usability and accessibility for touch-based devices.
Responsive Images and Media in Understanding Responsive Web Pages
Images create special challenges in responsive design, especially when considering responsive advertising. A high-resolution image perfect for desktops wastes bandwidth on mobile, while a small image looks pixelated on larger screens.
The most straightforward approach ensures images never overflow their containers:
img {
max-width: 100%;
height: auto;
}
But this doesn't solve the bandwidth problem; mobile users still download the full-sized image even though they see a smaller version. This creates the need to optimize images to ensure efficient performance across devices.
Basic Responsive Image Techniques
The object-fit property controls how images fill their containers, similar to background-size:
.card-image {
width: 100%;
height: 200px;
object-fit: cover; /* Crop to fill dimensions while maintaining aspect ratio */
}
This creates consistently-sized image containers without distortion. Images represent a substantial portion of website content, typically accounting for approximately 40-45% of a webpage's total byte size, making optimization essential for performance.
Properly optimized images are one of the most significant contributors to page weight. They are critical for maintaining fast loading times and a good user experience across all devices.
For CSS backgrounds, use media queries to serve different images based on screen size:
.hero {
background-image: url('hero-small.jpg');
}
@media (min-width: 768px) {
.hero {
background-image: url('hero-medium.jpg');
}
}
@media (min-width: 1200px) {
.hero {
background-image: url('hero-large.jpg');
}
}
Advanced Responsive Media Solutions
HTML5 introduced powerful tools for delivering the right image for each device:
The srcset attribute defines multiple image sources with width descriptors:
<img src="photo-800w.jpg"
srcset="photo-400w.jpg 400w,
photo-800w.jpg 800w,
photo-1600w.jpg 1600w"
sizes="(max-width: 600px) 100vw,
(max-width: 1200px) 50vw,
33vw"
alt="Responsive image">
This tells browsers which image to use based on screen size and resolution. The sizes attribute indicates how much viewport width the image will occupy at different breakpoints, helping browsers choose the optimal resource.
For art direction (showing different image crops rather than just different resolutions), use the picture element:
<picture>
<source media="(max-width: 600px)" srcset="photo-mobile.jpg">
<source media="(max-width: 1200px)" srcset="photo-tablet.jpg">
<img src="photo-desktop.jpg" alt="Responsive image">
</picture>
Some studies show that these techniques can reduce image bytes by 70-80% while maintaining visual quality across devices.
Understanding Typography in Responsive Web Pages
Responsive typography forms the backbone of most web content, yet it's often overlooked in responsive design, despite the importance of typography choices. Text that looks perfect on desktop can become impossible to read on mobile, highlighting the importance of typography in design, or waste space with too short lines, affecting mobile responsiveness.
Typography research suggests that the optimal line length for readability is 45-75 characters. This means adjusting font size and container width on smaller screens to maintain readability.
Using Relative Units for Scalable Text
Relative units create naturally responsive typography that scales smoothly across devices:
html {
font-size: 16px; /* Base size */
}
body {
font-size: 1rem;
line-height: 1.5;
}
h1 {
font-size: 2rem; /* 32px at default size */
}
@media (min-width: 768px) {
html {
font-size: 18px; /* Slightly larger base size on larger screens */
}
}
For more dynamic scaling that responds directly to viewport width, consider calc() and viewport units:
h1 {
font-size: calc(1.5rem + 1.5vw);
}
This creates headings that grow and shrink smoothly with the viewport, without requiring multiple breakpoints. The fixed rem component ensures text never becomes too small on mobile.
Creating Responsive Type Hierarchies
A proper type hierarchy helps users navigate content, but needs adjustment at different screen sizes:
/* Base styles (mobile) */
h1 {
font-size: 2rem;
margin-bottom: 1rem;
line-height: 1.2;
}
h2 {
font-size: 1.5rem;
margin-bottom: 0.75rem;
line-height: 1.3;
}
/* Adjusted for larger screens */
@media (min-width: 768px) {
h1 {
font-size: 2.5rem;
margin-bottom: 1.5rem;
}
h2 {
font-size: 1.75rem;
margin-bottom: 1rem;
}
}
The spacing between elements should increase on larger screens, as users typically view these displays from a greater distance.
Testing and Debugging Responsive Web Pages
Responsive design requires testing across devices and viewport sizes to ensure an enhanced user experience. Thankfully, modern development tools make this easier than keeping a drawer full of physical devices.
Test throughout development, not at the end. Those who test responsiveness continuously spend less time fixing issues than those who wait until the project's end.
Browser Tools for Responsive Testing
All modern browsers include device emulation tools in their developer consoles. In Chrome, Firefox, or Edge:
- Right-click on a page and select "Inspect" or press F12
- Click the device toggle icon (typically in the top toolbar)
- Select a preset device or drag handles to test custom dimensions
- Use the network throttling options to simulate slower connections
These tools let you see how your design responds across different screen sizes and network conditions. Chrome DevTools even supports device mode emulation features like touch simulation and device pixel ratio testing.
For more realistic testing, try dedicated services like BrowserStack or Sauce Labs that provide access to actual device environments rather than emulations.
Common Responsive Design Mistakes and Solutions
Watch for these frequent issues in responsive layouts:
Horizontal overflow causing unwanted scrollbars
/* Solution: Add to problematic containers */
.container {
overflow-x: hidden;
}
Touch targets too small for mobile
/* Solution: Ensure adequate tap area */
.button {
min-height: 44px;
min-width: 44px;
padding: 12px 16px;
}
Elements colliding at specific breakpoints
/* Solution: Add targeted spacing adjustment */
@media (min-width: 600px) and (max-width: 900px) {
.sidebar {
margin-right: 2rem;
}
}
Tables breaking layouts on small screens
/* Solution: Make tables horizontally scrollable */
.table-container {
overflow-x: auto;
-webkit-overflow-scrolling: touch; /* Smooth scrolling on iOS */
}
The latest Web Almanac report found that overlapping elements and unwanted horizontal scrolling are the most common responsive design bugs. These are usually caused by fixed-width elements or forgetting to add max-width to media.
Getting Help When Complexity Overwhelms
Responsive design involves juggling multiple technical considerations simultaneously, from fluid grids and flexible images to media queries and cross-device testing. If you're finding yourself overwhelmed by viewport units, breakpoint management, and debugging layout issues across different devices, you're not alone.
Many businesses discover that the time investment required to master responsive development often exceeds what their teams can realistically dedicate while maintaining other responsibilities.
When deadlines are tight and the technical learning curve feels steep, partnering with experienced web development professionals can be the most efficient path forward.
Teams like NoBoring Design specialize in handling the entire responsive development process using platforms like Webflow, managing everything from initial design concepts to cross-device testing and optimization. This approach lets you focus on your core business while ensuring your website delivers exceptional experiences across all devices without the technical headaches.
Your Path Forward with Understanding Responsive Web Pages
This step-by-step approach prevents overwhelm and makes testing manageable. Begin with the viewport meta tag and fluid containers, then add more advanced techniques as you go.
Creating truly responsive web pages requires technical know-how and design sensibility. To stay ahead of responsive design trends, focus on experiences that feel natural on each device rather than just shrinking a desktop design.
When your content is accessible, readable, and usable regardless of screen size, you've created a genuinely responsive web experience that works for everyone.
Ready to bring your responsive design vision to life without the complexity? No Boring Design combines technical expertise with creative flair to deliver web designs that work beautifully across every device. Reach out today!
Key Takeaways
- The viewport meta tag establishes the foundation for mobile rendering.
- Fluid layouts using relative units create flexibility across screen sizes.
- Modern layout methods like Flexbox and Grid simplify responsive implementations.
- Media queries enable targeted style adjustments for different devices.
- Responsive images and video deliver appropriate resources to each device.
- Typography needs thoughtful scaling to maintain readability everywhere.
FAQ
We have the answers.
Responsive web pages automatically adapt their layout and content to different screen sizes and devices. They're essential for providing optimal user experiences across mobile, tablet, and desktop devices while improving search rankings and accessibility.
Make websites responsive using fluid layouts with relative units, CSS Grid and Flexbox for flexible structures, media queries for device-specific styling, and the viewport meta tag to ensure proper mobile rendering and scaling.
Mobile-first design starts with mobile layouts and progressively enhances for larger screens, while responsive design adapts existing layouts. Mobile-first typically creates more efficient code, better mobile performance, and improved user experiences.
Key responsive design elements include the viewport meta tag, fluid grids using percentages, flexible images with max-width properties, media queries for breakpoints, and scalable typography using relative units like rem and em.